6 min to read
Kubernetes Operators and Custom Resource Definitions (CRDs)
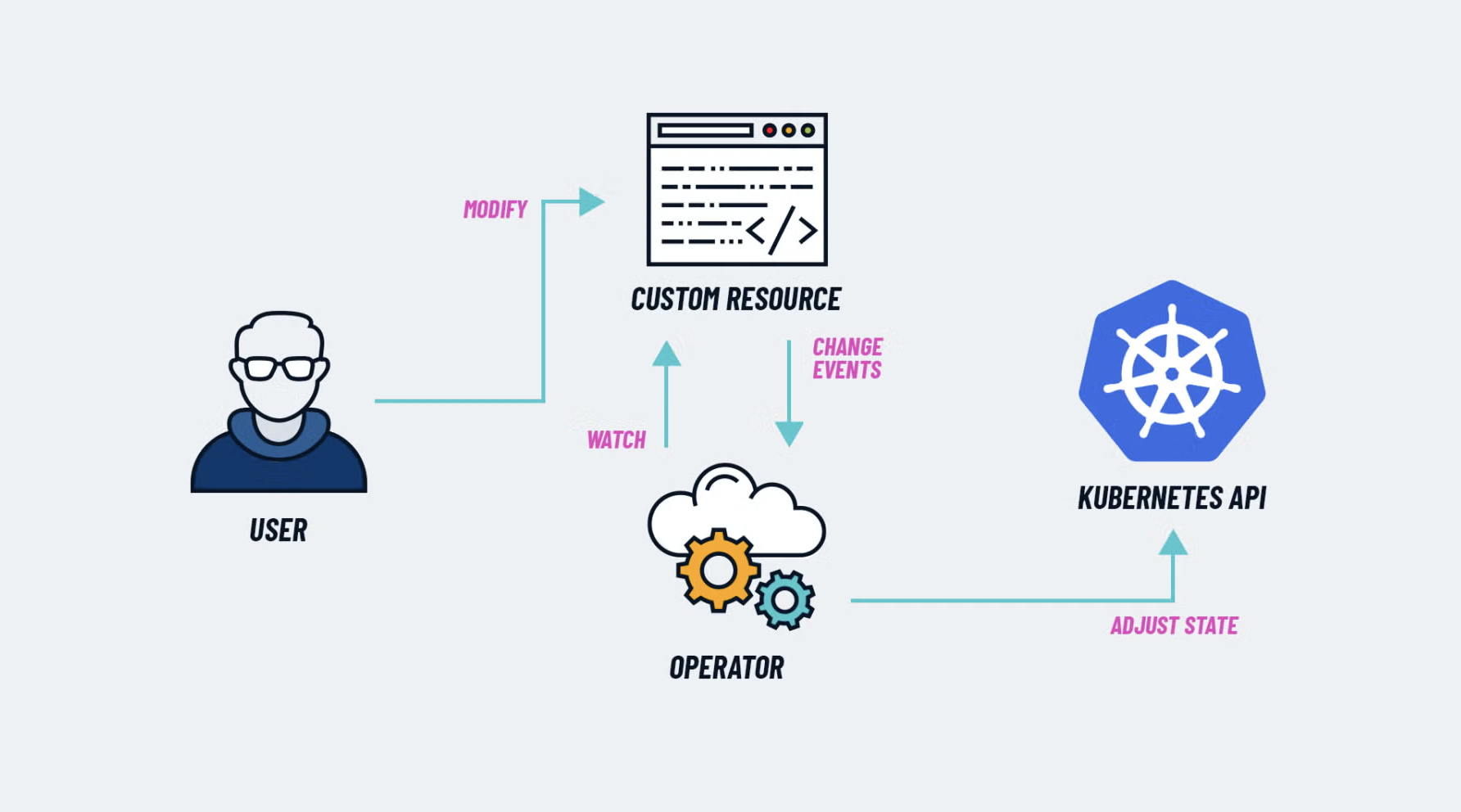
Overview
Understanding Kubernetes Operators and Custom Resource Definitions (CRDs), essential components for extending Kubernetes functionality.
Kubernetes applications are distributed to Kubernetes and managed using the Kubernetes API and kubectl tools.
The Operator follows the Kubernetes principle of the controller, a loop that monitors cluster health and then makes changes or requests, if necessary.
The Operator expands Kubernetes to automate the full life cycle management of a particular application.
Kubernetes Operator Basics
What is an Operator?
- Custom controller that extends Kubernetes functionality
- Automates application lifecycle management
- Implements domain-specific operational knowledge
- Follows Kubernetes control loop principles
Why Use Operators?
- Automation
- Application deployment
- Configuration management
- Updates and upgrades
- Backup and recovery
- Consistency
- Standardized operations
- Reduced human error
- Repeatable processes
Manage life cycles
Operator uses Kubernetes’ concept of control loop to manage applications. Application-specific actions are taken to continuously monitor the state of the application and to correct inconsistencies with the desired state.
- Automated deployment of applications and potential support services
- Seamlessly handle upgrades and downgrades, including complex stateful applications
- Application configuration and confidentiality management
- Automatically scale based on load or other metrics
- Error recovery, automatic replacement or reconfiguration of abnormal instances
- Backup and Restore
Operator development
There are frameworks and tools for developing operators.
- Operator SDK (Operator SDK): Help develop, test, and package operators.
- Operator Lifecycle Manager (OLM): It manages operators on the Kubernetes cluster, and is responsible for installing, updating, and life cycle management of operators.
- Operator Metering: This is for reporting on the resources used by the operator.
Custom Resource Definitions (CRDs)
What is CRD?
CRD allows you to create specific resources that are unique within a Kubernetes cluster in a manner similar to how standard Kubernetes resources, such as fords, deployments, or services, are handled.
This can be very useful in developing custom applications or integrations based on the Kubernetes platform.
What is Custom Resource?
This is basically a method of customizing Kubernetes to meet your needs by adding new resources in addition to the resources provided.
Why do I use CRD?
- Extensibility: Kubernetes can be extended to their own APIs that can be used with Kuberectl and other Kubernetes API clients.
- Flexibility: You can define new resources that act like basic Kubernetes resources.
- Integration: CRD is useful for building operators, which are custom controllers that manage applications and their components based on custom resources.
How does CRD work?
- To implement CRD, as with other Kubernetes resources, it is defined using YAML. This definition specifies a new kind of resource, its name, and schema. The schema is used to validate the configuration of a CRD (also known as a custom resource).
- The basic structure of the CRD definition is as follows.
Basic CRD Example
apiVersion: apiextensions.k8s.io/v1
kind: CustomResourceDefinition
metadata:
name: crdtype.mycompany.com
spec:
group: mycompany.com
versions:
- name: v1
served: true
storage: true
schema:
openAPIV3Schema:
type: object
properties:
spec:
type: object
properties:
myField:
type: string
scope: Namespaced
names:
plural: crdtypes
singular: crdtype
kind: CrdType
shortNames:
- ct
Lifecycle and Controllers
- After you define and apply CRD in the cluster, you can create and manage that instance, just like any other resource in Kubernetes.
- The following is a basic example of a user-defined resource.
apiVersion: mycompany.com/v1
kind: CrdType
metadata:
name: example-crdtype
spec:
myField: "Hello, world!"
- In general, CRD is part of an operator consisting of the CRD itself and a user-defined controller. The controller monitors events related to custom resources and responds by creating, updating, deleting, or coordinating resources accordingly.
Kubebuilder Framework
Kubbuilder is an SDK for developing Kubernetes Operator. It is a framework that makes it easy to develop CRD and controllers using the Go language.
Project Structure
.
├── Dockerfile
├── Makefile # Build, test, deploy commands
├── PROJECT # Project metadata
├── api/ # CRD API definitions
│ └── v1/
├── config/ # Kubernetes manifests
│ ├── crd/
│ ├── rbac/
│ └── manager/
└── main.go # Entry point
Common Commands
# Initialize new project
kubebuilder init --domain example.com
# Create API
kubebuilder create api --group sync --version v1 --kind MyResource
# Build and deploy
make install
make run
make docker-build
make deploy
Development Workflow
Stage | Activities |
---|---|
Design |
- Define CRD specs - Plan controller logic - Design API schema |
Implementation |
- Write controller code - Implement reconciliation - Add validation |
Testing |
- Unit tests - Integration tests - E2E testing |
Deployment |
- Build container image - Deploy to cluster - Monitor performance |
Best Practices
1. API Design: - Use semantic versioning
- Include validation rules
- Document fields properly
2. Controller Implementation: - Handle errors gracefully
- Implement proper logging
- Use status subresource
3. Testing: - Write comprehensive tests
- Use test fixtures
- Mock external dependencies
4. Deployment: - Use proper RBAC
- Monitor resource usage
- Implement graceful shutdown
Comments