9 min to read
Understanding Kubernetes Resources - A Comprehensive Guide
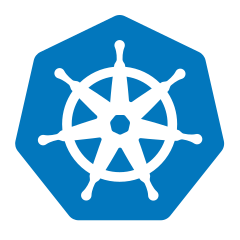
🎯 Overview
Kubernetes offers various resource types to manage different aspects of container orchestration. Let’s explore each type in detail.
Kubernetes Resources
- Deployments
- ReplicaSet
- StatefulSets
- DaemonSet
- Jobs
- Automatic Cleanup for Finished Jobs
- CronJob
- ReplicationController
🔄 Deployment & ReplicaSet & Pod
1. ReplicaSet
ReplicaSet is a resource that allows the desired number of replicas (instances) of a Pod to run at a specified time.
If the number of running pods is less than the desired number, ReplicaSet creates a new pod to maintain the desired number.
Similarly, if there are too many Pods running, ReplicaSet will terminate the additional Pods.
ReplicaSet operates based on the Pod template that defines the desired state of the Pod managed by ReplicaSet.
2. Deployment
Deployment is a resource that manages the desired state of an application.
This allows you to define the number of copies of containerized applications you want to run at a given time and manages updates for applications such as Rolling Update or Rollback.
Deployment uses ReplicaSet to manage the basic pods that configure applications.
# Example Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: nginx-deployment
spec:
replicas: 3
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx:1.14.2
- Replicaset occurs automatically when you apply Deployment yaml as above.
- In other words, it can be seen that replicaset is included in the deployment.
Rolling Updates
# Update image
kubectl set image deployment/nginx-deployment nginx=nginx:1.16.1
# Check status
kubectl rollout status deployment/nginx-deployment
# View history
kubectl rollout history deployment/nginx-deployment
# Rollback
kubectl rollout undo deployment/nginx-deployment
Checking Rollout History of a Deployment
The Rollout method is the same as the method of Rolling Update above.
Rollout is a way to update multiple pods sequentially without killing them all!
–Revision occurs in the case of deployments that are applied with the --record
option.
Alternatively, you can change the image using the set image
command, and the revision occurs.
Therefore, I would like to find out how to check the Rollout History and how to return it.
kubectl rollout history deployment/nginx-deployment
deployments "nginx-deployment"
REVISION CHANGE-CAUSE
1 kubectl apply --filename=https://k8s.io/examples/controllers/nginx-deployment.yaml
2 kubectl set image deployment/nginx-deployment nginx=nginx:1.16.1
3 kubectl set image deployment/nginx-deployment nginx=nginx:1.161
# Rollback to desired version
kubectl rollout history deployment/nginx-deployment --revision=2
# Rollback to previous version
kubectl rollout undo deployment/nginx-deployment
3. Pod
Pod is the smallest and most basic unit in the Kubernetes object model.
It represents a single instance of an application running in the cluster and consists of one or more containers closely coupled to each other. Containers within the Pod share the same network namespace and can communicate with each other using
‘localhost’. Even if the Pod fails, it is not automatically re-booked on another node. ReplicaSet operates to maintain the desired number of Pods.
🌐 DaemonSet & StatefulSet
1. DaemonSet
DaemonSet is a Kubernetes resource that allows certain Pod to run on all (or subset) nodes in the cluster.
Designed to run a single pod on each worker node, i.e. the daemonset pod cannot be extended on the node.
And for some reason, if the daemonset pod is deleted from the node, the daemonset controller generates it again.
It is useful for deploying system-level services such as log collectors, monitoring agents, or networking components that must be run on each node for proper functionality.
k get daemonset -n kube-system
NAME DESIRED CURRENT READY UP-TO-DATE AVAILABLE NODE SELECTOR AGE
calico-node 6 6 6 6 6 kubernetes.io/os=linux 364d
kube-proxy 6 6 6 6 6 kubernetes.io/os=linux 364d
nodelocaldns 6 6 6 6 6 kubernetes.io/os=linux 364d
apiVersion: apps/v1
kind: DaemonSet
metadata:
name: fluentd-elasticsearch
spec:
selector:
matchLabels:
name: fluentd-elasticsearch
template:
metadata:
labels:
name: fluentd-elasticsearch
spec:
containers:
- name: fluentd-elasticsearch
image: quay.io/fluentd_elasticsearch/fluentd:v2.5.2
How Daemon Pods are scheduled?
So how will Daemonset pod be scheduled?
As shown in the figure above, it is scheduled in consideration of NodeSelector, NodeAffinity, Priority Class, Taints & Tolerations.
2. StatefulSet
StatefulSet is a Kubernetes resource that manages a set of Pods that are based on a specific template and have a stable network identity and persistent storage.
StatefulSet is a Kubernetes resource that manages the deployment and expansion of state-stored applications, i.e., applications that require stable network IDs and persistent storage.
Ensure that each Pod generated obtains a unique and stable hostname such as web-0 and web-1 based on the index.
This makes it easier to manage applications that require stable network IDs or rely on deployment and expansion sequences.
StatefulSet also provides guarantees of the order and uniqueness of pods that are essential for state-stored applications such as databases or distributed systems.
It can be used with persistent storage, so that data associated with a particular Pod remains in that Pod even if it is rescheduled to another node.
It is used to distribute state-stored applications such as Redis, databases such as PostgreSQL, MySQL, or MongoDB, and message queues such as RabbitMQ.
apiVersion: apps/v1
kind: StatefulSet
metadata:
name: web
spec:
serviceName: "nginx"
replicas: 3
selector:
matchLabels:
app: nginx
template:
metadata:
labels:
app: nginx
spec:
containers:
- name: nginx
image: nginx
volumeMounts:
- name: www
mountPath: /usr/share/nginx/html
⏱️ Jobs & CronJobs
1. Job
Job is a Kubernetes resource that manages a one-time or recurring task.
It is used to run tasks that are not intended to run indefinitely, such as data processing, batch jobs, or one-time tasks.
One or more pods are created to perform the specified task and the pod ends when the job is complete.
You can use a job for tasks such as data processing, batch processing, or one-time script or command execution.
Job tracks the overall progress and status of the task to ensure that a specified number of completions have been achieved.
If the Pod fails or is removed due to resource constraints, Job creates a new Pod to replace the Pod and tries the task again until it is successfully completed or Job reaches the backoff limit.
# Example Job
apiVersion: batch/v1
kind: Job
metadata:
name: hello-job
spec:
template:
spec:
containers:
- name: hello
image: busybox
2. CronJob
CronJob is a Kubernetes resource that allows you to schedule recurring tasks.
For example, you can easily prepare a backup creation strategy or get old resources. It is generally useful for applications that require periodic execution of certain tasks.
The main difference between Kubernetes CronJob and Linux is the use of containers.
Linux CronJob runs as part of the base system (OS), gaining access to all important services, sharing resources and file systems, and using Kubernetes, all operations occur within the pod. That means that not only are the jobs isolated and have dedicated resources, but also inherit all the functions of other Kubernetes pods, so you can run multiple containers within a single pod if you want.
# Example CronJob
apiVersion: batch/v1
kind: CronJob
metadata:
name: hello-cronjob
spec:
schedule: "*/10 * * * *"
jobTemplate:
spec:
template:
spec:
containers:
- name: hello
image: busybox
command: ["/bin/sh", "-c", "date"]
restartPolicy: OnFailure
📦 Kubernetes Workload Resources Comparison
🔧 Feature | 🚀 Deployment | 📦 StatefulSet | 🛠️ DaemonSet | ⏳ Job/CronJob |
---|---|---|---|---|
Purpose | Stateless apps | Stateful apps | Node-level operations | Batch tasks |
Scaling | Dynamic | Ordered | Node-based | Task-based |
Storage | Optional | Persistent | Optional | Temporary |
Naming | Random | Predictable | Node-based | Random |
Updates | Rolling | Ordered | Rolling | N/A |
💡 Key Points
1. Deployment
- Manages ReplicaSets
- Supports rolling updates
- Best for stateless applications
2. StatefulSet
- Stable network identities
- Ordered deployment
- Persistent storage
3. DaemonSet
- One pod per node
- System-level operations
- Node monitoring
4. Jobs/CronJobs
- Batch processing
- Scheduled tasks
- One-time operations
Comments